The next blog post is just a fun post which shows how you can totally mess up the intellisense in Visual Studio.
Result
So what do we want to achieve in this recipe?
Have you ever seen your intellisense displaying a list like this one?

No, dear reader, you are not drunk: FirstName and LastName are displayed twice in the intellisense suggestions.
Let’s see how we can produce this strange behavior.
Step 1
Start by creating two classes that both have a FirstName and a LastName property. In this case I created an Employee and a Person class. The Employee class also has a MiddleName property.
All properties are of type string and the Employee and Person class are in the same namespace.
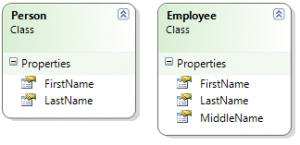
Step 2
Create a (static) class which has two methods with the same name. Both methods expect one parameter of a generic Func type (or a delegate) which returns a string. The first method wants a first parameter of type Person, the other one expects an Employee as first parameter.
I ended up with the following static MessUp class:
public static class MessUp { public static string HereYouGo(Func<Person, string> pFunction) { //Do something here } public static string HereYouGo(Func<Employee, string> eFunction) { //Do something here } }
Step 3
The only thing we now have to do is call the HereYouGo method on the MessUp class (or whatever you called it) and pass the generic Action with a lambda expression.
So start with typing something like this:
MessUp.HereYouGo(whoAmI => whoAmI.)
If you arrive at the dot after whoAmI, the compiler still doesn’t know which method it needs to call: both methods have the same name and at the moment of typing the dot to address a property of Employee or Person, the compiler has no clue which type you want to use, so it just displays all the properties of both types, which is pretty weird off course!
If you select the FirstName property, you cannot compile this code, because the compiler doesn’t know which FirstName property (the Employee.FirstName or the Person.FirstName) it must use.

So how can we produce a work around for this issue?
- Use property names which are not present in both classes: in this case the Employee.MiddleName property will not pose any problem for the compiler.
- Define a strong typed method in which you fetch the desired property:
private static string EmployeeFirstName(Employee employee) { return employee.FirstName; }
Then you can just rewrite the code like this (no lambda expression needed):
MessUp.HereYouGo(EmployeeFirstName);